OPINION
What is Version Control in Software?
October 4, 2024 • by digitalproductsdp.com
Share on
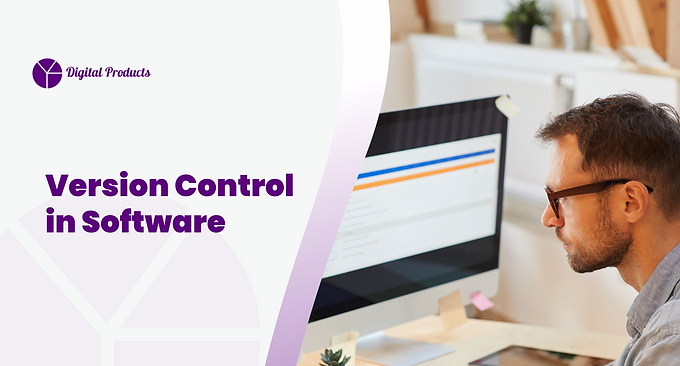
Version control is a way to track changes in software code over time. Version control systems help developers manage different versions of their code, collaborate with others, and maintain a history of project changes.
This practice allows teams to work on the same codebase without overwriting each other's work. These systems save snapshots of files at different points in time. This lets developers revert to earlier versions if needed, compare changes, and merge work from other team members.
What is Version Control?
Version control is a system that tracks changes to files over time. It lets developers save different versions and go back to earlier ones if needed. Version control also helps teams work together on code.
Definition and Concepts
Version control is a way to manage changes to computer files, especially code. It keeps a record of who made what changes and when. This makes it easier to fix mistakes and compare different versions.
There are two main types of version control systems. Centralized systems store all files on one server. Distributed systems let each developer have a full copy of the project.
Version control tools offer many benefits. They allow multiple people to work on the same project without overwriting each other's changes. Developers can create separate branches to test new ideas without affecting the main code.
Types of Version Control Systems
Version control systems help developers manage code changes over time. There are three main types:
Local Version Control Systems
Centralized Version Control Systems
Distributed Version Control Systems
Local systems store file versions on a single computer. They're simple but limit collaboration.
Centralized version control systems use one main server to hold all files. Developers check out and commit changes to this central repository. This setup makes sharing easier but relies on server availability.
Distributed systems like Git give each developer a full copy of the repository. This allows for offline work and better backup options. Here's a quick comparison:
Type | Collaboration | Offline Work | Server Dependency |
Local | Limited | Yes | No |
Centralized | Good | No | High |
Distributed | Excellent | Yes | Low |
Each type has its pros and cons. The choice depends on project size, team location, and workflow needs. Modern software development often uses distributed systems. They offer flexibility and robust collaboration features.
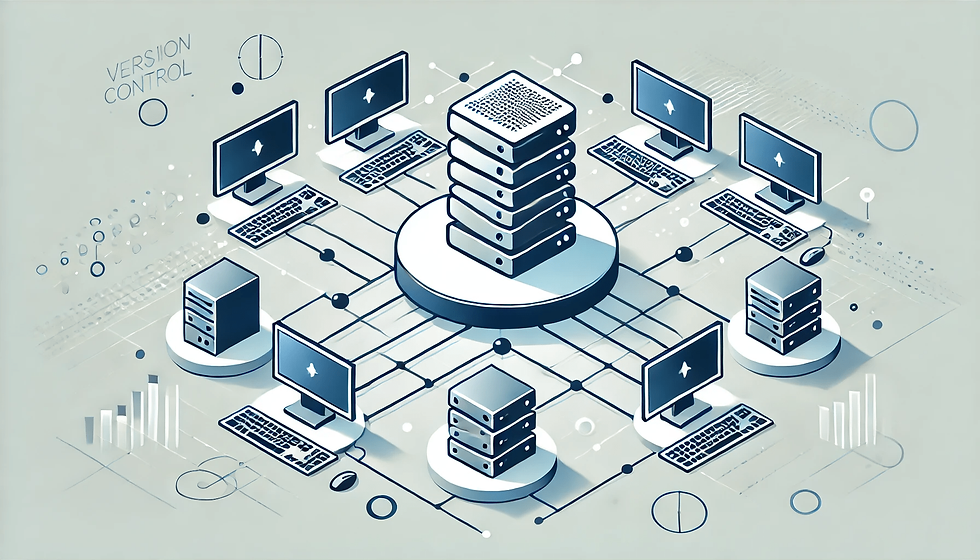
Version Control Systems and Tools
Version control systems help developers track and manage code changes. They offer different features and workflows to suit various project needs.
Git
Git is a distributed version control system that's widely used in software development. It allows multiple developers to work on the same project simultaneously.
Git stores snapshots of files over time. This lets developers easily track changes and revert to previous versions if needed. It also supports branching, which allows for parallel development of features.
One of Git's strengths is its speed and efficiency. It handles large projects well and works offline. Git's popularity has led to many hosting platforms like GitHub and GitLab.
Subversion (SVN)
Subversion, or SVN, is a centralized version control system. Unlike Git, it uses a single central repository that developers connect to.
SVN tracks file and directory changes over time. It allows users to recover older versions of data or examine the history of how data changed.
One advantage of SVN is its simplicity. It's often easier for beginners to understand than distributed systems. SVN also handles large files well, making it useful for projects with many binary assets.
Mercurial
Mercurial is another distributed version control system. It's known for its simplicity and ease of use. Like Git, it allows for offline work and supports branching.
Mercurial uses a command-line interface but also has graphical tools available. It's designed to handle both small and large projects efficiently.
One of Mercurial's strengths is its strong safeguards against data corruption. It also has built-in web interface capabilities, making it easy to share repositories.
Perforce
Perforce is a commercial version control system often used in large-scale development. It's known for its ability to handle very large codebases and binary files.
Perforce uses a client-server model. It offers strong performance even with many users and large file sizes. This makes it popular in industries like game development.
One unique feature of Perforce is its "shelving" capability. This allows developers to temporarily store changes without committing them. Perforce also offers robust branching and merging tools.
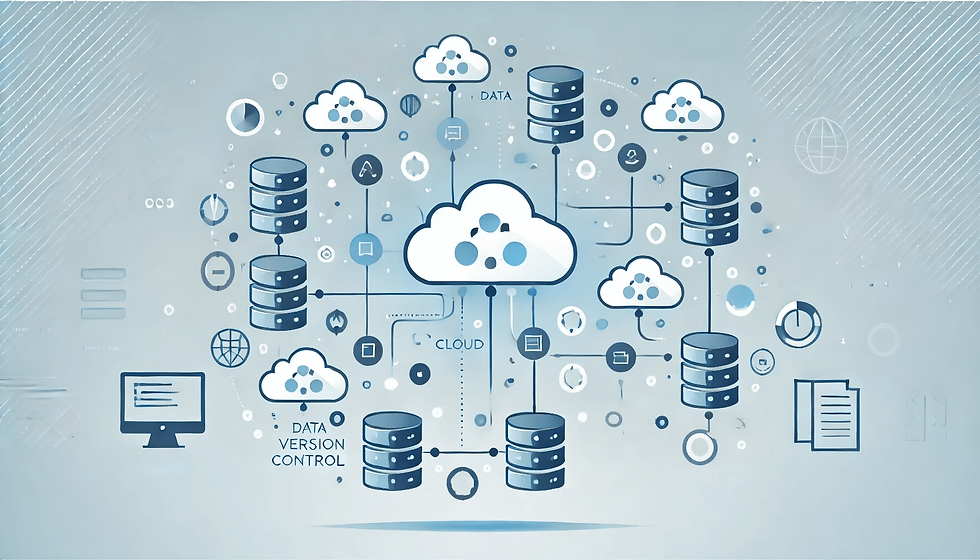
Working with Repositories
Repositories are central to version control systems. They store code, track changes, and enable collaboration. Let's explore how to set up and use repositories effectively.
Initialization
To start a new project with version control, you need to initialize a repository. This creates a hidden folder to track changes. For Git, use the command "git init" in your project directory. This sets up the basic structure for version control.
After initialization, add files to the repository. Use "git add" to stage changes and "git commit" to save them. This creates a snapshot of your project at that point in time.
Remember to create a .gitignore file. It tells Git which files to ignore, like temporary files or build outputs.
Cloning
Cloning creates a copy of an existing repository. It downloads all files and history to your local machine.
To clone a repository, use the "git clone" command followed by the repository URL. This creates a new folder with the project files. Cloning is useful for:
Starting work on an existing project
Creating a backup of a repository
Setting up a new development environment
After cloning, you can make changes, commit them, and push updates back to the original repository if you have permission.
Forking
Forking is similar to cloning, but it happens on the server side. It creates a separate copy of a repository under your account. Forking is common in open-source projects. It allows you to:
Experiment with changes without affecting the original project
Propose improvements through pull requests
Create a new project based on existing code
To fork a repository, use the "Fork" button on platforms like GitHub or GitLab. Then clone your forked version to work on it locally. When you're ready to share changes, create a pull request. This asks the original project to consider your updates.

Change Management
Version control systems handle changes to code over time. They let teams track modifications, undo mistakes, and work together smoothly.
Commits
Commits are snapshots of code at a specific point. Each commit has a unique ID and message describing the changes. Developers make commits when they finish a task or reach a milestone.
Version control systems store the full history of commits. This allows teams to see who changed what and when. Commits can be compared to spot differences between versions.
Good commit messages help others understand the changes. They should be clear and concise. For example:
"Fix login bug"
"Add new user profile page"
"Update API documentation"
Merge vs Rebase
Merging and rebasing are two ways to combine code changes. Merging creates a new commit that joins two branches. Rebasing moves commits to a new base.
Merging is simpler but can clutter history. It's good for integrating feature branches. Rebasing gives a cleaner history but can be trickier to use. It works well for updating a branch with the latest changes.
The choice depends on the team's workflow. Some prefer merging for its simplicity. Others like rebasing for a linear history.
Conflict Resolution
Conflicts happen when two changes can't be combined automatically. This often occurs when merging or rebasing branches. Resolving conflicts requires manual intervention.
Most version control tools highlight conflicting sections. Developers then choose which changes to keep. They might need to edit the code to blend both changes.
Communication is important during conflict resolution. Team members should discuss the best approach. This helps ensure the final code meets everyone's needs.
Version control systems track how conflicts are resolved. This information can be useful if similar issues arise later.
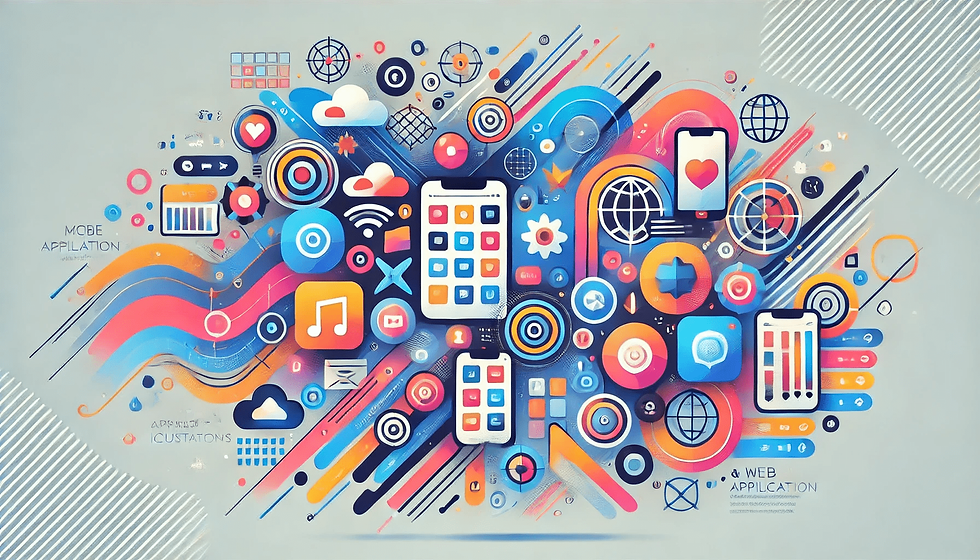
Branching Strategies
Branching strategies help teams manage code changes and collaborate effectively. They set guidelines for creating and merging different code versions.
Feature Branch Workflow
The feature branch workflow keeps new development separate from the main codebase. Developers create a new branch for each feature or bug fix. This allows work to happen in parallel without affecting the main branch.
When a feature is ready, the developer opens a pull request. Team members can then review the code and suggest changes. Once approved, the feature branch gets merged back into the main branch.
This approach helps catch bugs early and promotes code quality. It also makes it easier to track which changes are associated with specific features.
Gitflow Workflow
Gitflow is a branching model that uses multiple branches for development, features, and releases. It has two main branches: master and develop.
The master branch holds production-ready code. The develop branch is for ongoing work and integrating features.
Feature branches split off from develop. When complete, they merge back into develop. Release branches prepare new versions, allowing last-minute fixes.
Hotfix branches address critical bugs in production. They split from master and merge back into both master and develop. Gitflow works well for scheduled releases but can be complex for continuous delivery.
Forking Workflow
The forking workflow is common in open-source projects. It gives each developer their server-side repository. To start, a developer forks the official repository. This creates a personal copy on the server. The developer then clones their fork to work locally.
When ready to share, the developer pushes changes to their public fork. They then open a pull request to the official repository.
This method reduces friction for new contributors. It also gives project maintainers full control over what gets merged.
The forking workflow scales well for large, distributed teams. But it can lead to many divergent copies of the codebase.
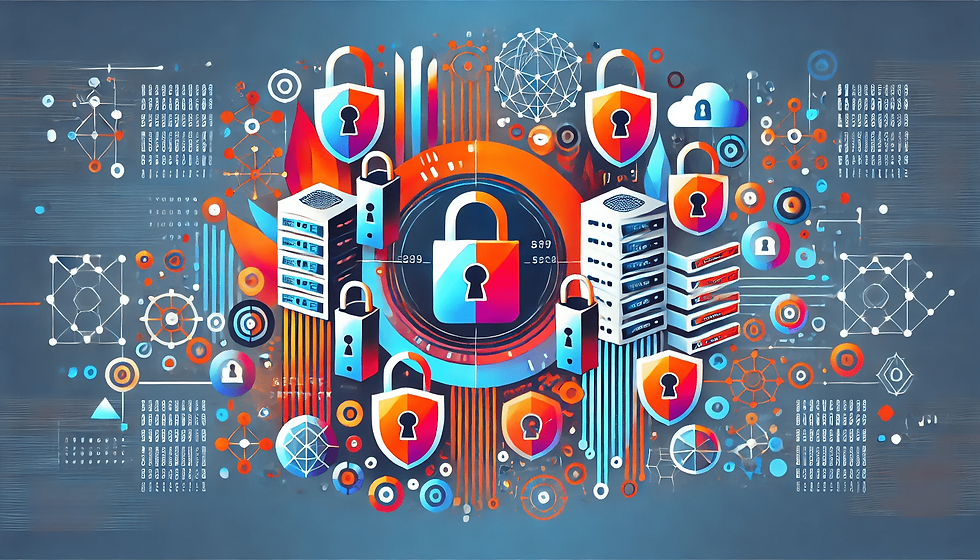
Collaborative Development
Version control systems enable teams to work together on code. They provide tools for sharing changes, reviewing code, and programming in pairs.
Pull Requests
Pull requests let developers propose changes to a codebase. A developer creates a branch, makes changes, and opens a pull request to merge those changes back into the main branch.
This process allows other team members to review the changes before they are added. Pull requests often include descriptions of the changes, why they were made, and any related issues or tasks.
Many version control systems have built-in tools for pull requests. These tools show diffs, allow comments, and track the status of the review process.
Code Reviews
Code reviews are a way for developers to check each other's work. A reviewer looks at proposed changes and gives feedback.
Reviews help catch bugs, improve code quality, and share knowledge among team members. They can happen through pull request comments or dedicated code review tools. Good code reviews focus on:
Code correctness
Design and architecture
Test coverage
Documentation
Coding standards
Pair Programming
Pair programming involves two developers working together on the same code. One person types (the "driver") while the other watches and gives input (the "navigator"). This technique can:
Improve code quality
Reduce bugs
Help share knowledge
Boost team communication
Pairs switch roles often to keep both developers engaged. Some version control systems have features to support pair programming, like shared editing sessions.
Pair programming works well for complex problems or when onboarding new team members. It can be done in person or remotely using screen sharing tools.
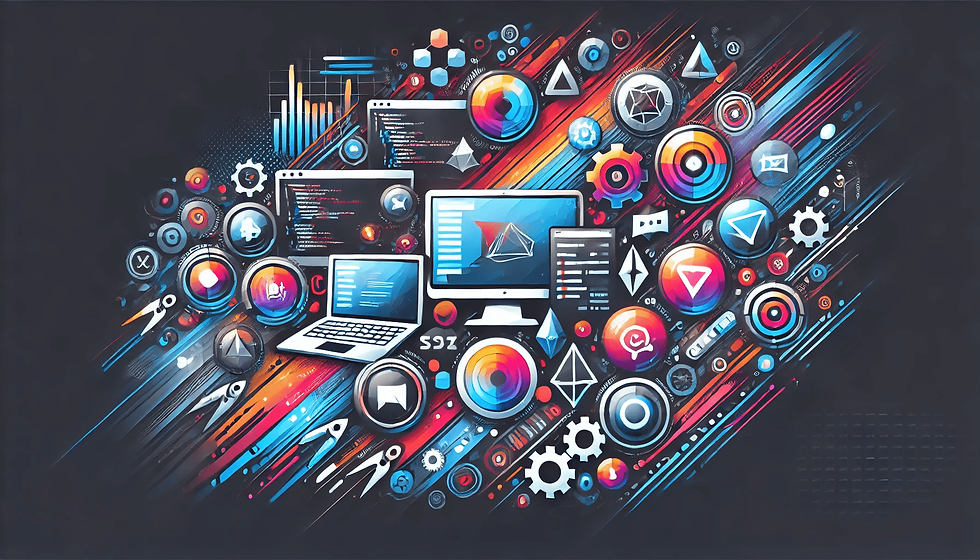
Version Control Best Practices
Good version control habits help teams work together smoothly and keep code organized. These practices make it easier to track changes, fix bugs, and release new features.
Commit Guidelines
Clear and frequent commits are important for effective version control. Write short, descriptive commit messages that explain what changed and why. Aim for one feature or fix per commit.
Use present tense verbs in commit messages. For example: "Add login feature" or "Fix payment bug." This makes the history easier to read.
Group related changes into separate commits. This helps when reviewing code or rolling back specific features. It's better to make many small commits than a few large ones.
Branch Organization
A well-organized branch structure keeps development tidy. Use a main branch for stable code and create feature branches for new work.
Name branches clearly to show their purpose. For example: "feature/user-authentication" or "bugfix/checkout-error".
Merge feature branches into the main branch once they're complete and tested. Delete old branches after merging to avoid clutter.
Some teams use long-lived branches for different stages, like development, staging, and production. This can help manage releases.
Version Tagging
Version tags mark important points in a project's history. They're usually used for releases or major milestones. Use semantic versioning (SemVer) for clear version numbers. This system uses three numbers: major.minor.patch. For example: 2.1.3.
Increase the major version for big changes, minor for new features, and patch for bug fixes. This helps users understand the impact of updates.
Add descriptive notes to each tagged version. List new features, bug fixes, and any breaking changes. This creates a helpful changelog for users and developers.
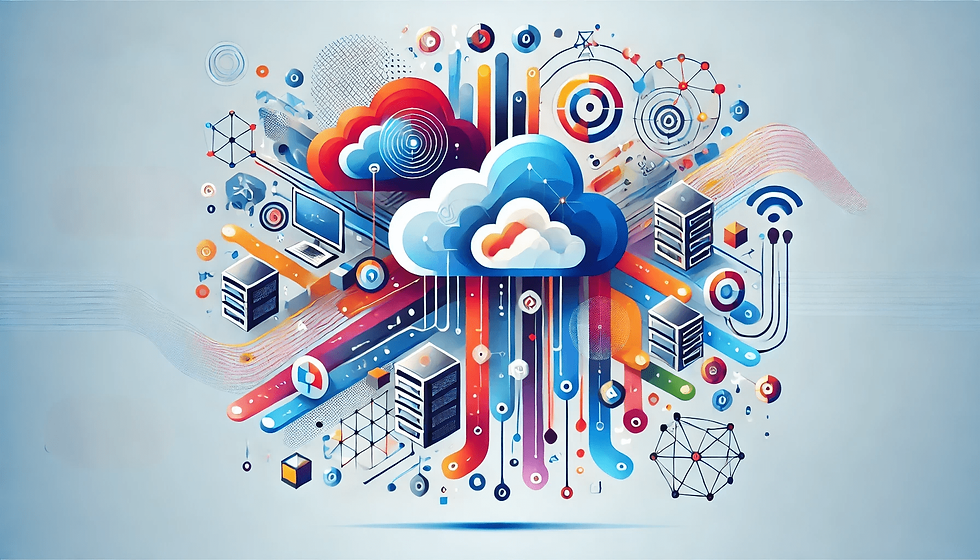
Security in Version Control
Version control systems need strong security measures to protect code and data. These systems use access control, secure transmission, and encrypted storage to keep information safe.
Access Control
Multi-factor authentication helps secure version control systems. It requires users to provide two or more pieces of evidence to log in. This can include passwords, security tokens, or biometric data.
Role-based access control limits what users can do in the system. Admins can set permissions for different roles. For example, some users might only be able to view code, while others can make changes.
Regular audits of user access are important. This helps catch any unauthorized users or outdated permissions. Removing access for former team members quickly is also a good practice.
Secure Transmission
Encryption protects data as it moves between users and servers. HTTPS is a common protocol for secure web connections. It uses TLS to encrypt data in transit.
SSH (Secure Shell) is often used for Git operations. It provides a secure channel for remote logins and file transfers. SSH keys offer a more secure alternative to passwords for authentication.
Virtual Private Networks (VPNs) can add an extra layer of security. They create an encrypted tunnel for all internet traffic, including version control operations.
Encrypted Storage
Encrypting data at rest protects it from unauthorized access. This applies to both the main repository and any backups. Strong encryption algorithms like AES are commonly used.
Password managers can help users create and store complex encryption keys. This reduces the risk of weak keys being used. Some version control systems offer built-in encryption features. Others may require third-party tools or plugins. It's important to choose a solution that fits your security needs.
Regular software updates are critical for maintaining strong encryption. They often include fixes for security vulnerabilities.
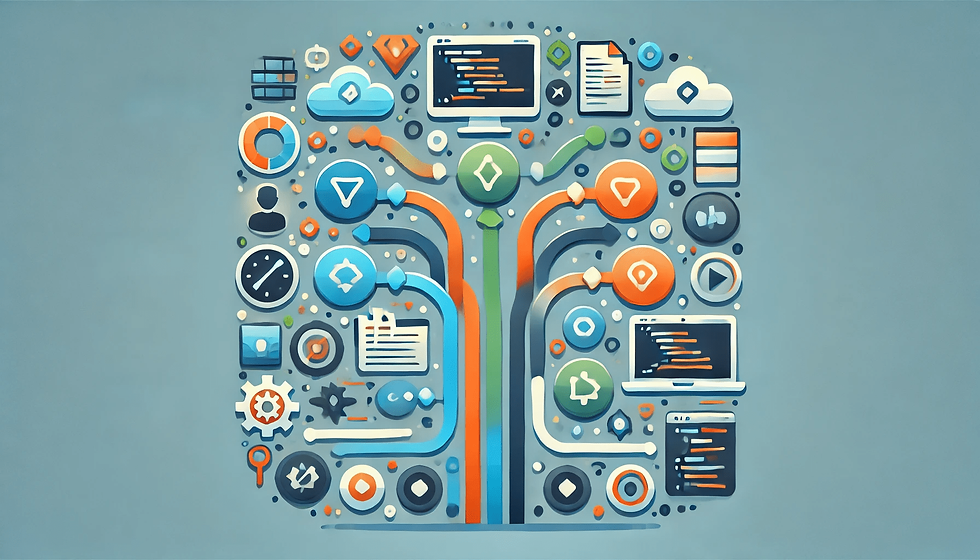
Continuous Integration/Continuous Deployment (CI/CD)
CI/CD practices streamline software development by automating code integration, testing, and deployment. This approach speeds up release cycles and improves software quality.
Automated Testing
CI/CD relies on automated testing to catch bugs early. Tests run automatically when developers push code changes. This includes unit tests, integration tests, and end-to-end tests.
Automated tests check if new code works with existing features. They also verify that changes don't break other parts of the software. This helps teams find and fix issues quickly.
Many tools support automated testing in CI/CD pipelines. Popular options include Jenkins, GitLab CI, and Travis CI. These tools integrate with version control systems and run tests on every commit.
Build Automation
Build automation is a core part of CI/CD. It compiles source code, packages applications, and prepares them for deployment. This process happens automatically when developers push changes.
Automated builds ensure consistency across different environments. They reduce human error and save time. Build scripts define the steps needed to create deployable artifacts.
Common build automation tools include Maven, Gradle, and npm. These tools manage dependencies and create standardized build processes. They work with CI/CD platforms to trigger builds on code changes.
Deployment Automation
Deployment automation moves code from development to production environments. It reduces manual steps and improves reliability. Automated business monitoring can help track the success of deployments.
Deployment scripts handle tasks like database migrations and configuration updates. They also manage rolling updates to minimize downtime. Automation tools can roll back changes if problems occur.
Popular deployment automation tools include Ansible, Puppet, and Kubernetes. These tools manage infrastructure and application deployments. They integrate with CI/CD pipelines to enable continuous deployment.
Deployment automation also includes monitoring and alerting. This helps teams quickly respond to issues in production environments.
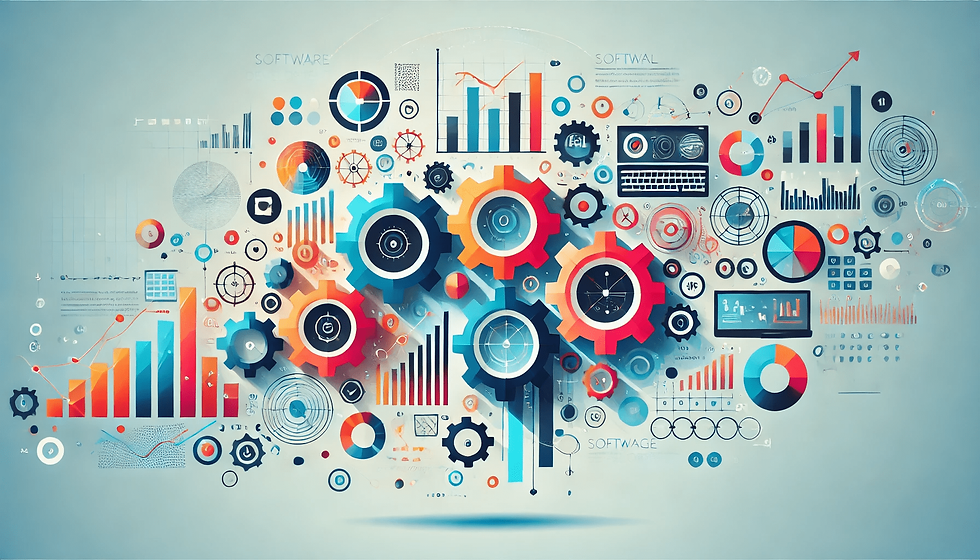
Scaling Version Control
Version control systems need to adapt as projects grow larger and more complex. Bigger codebases, performance concerns, and distributed teams all present challenges.
Handling Large Codebases
As software projects expand, version control must keep up. Big codebases can slow down operations like cloning and fetching. To address this, many teams use shallow clones. These fetch only recent history, reducing download times.
Another approach is splitting repositories. This breaks a large project into smaller, more manageable parts. Each part becomes its own repository. This makes cloning and working with code faster.
Some version control systems offer specialized features for big projects. For example, Git has partial clones and sparse checkouts. These let developers work with only the parts of the code they need.
Performance Optimization
As projects scale, maintaining good performance becomes harder. Slow version control can hurt productivity. There are several ways to speed things up.
Local operations should be fast. This includes committing changes and switching branches. Good indexing helps here. It lets the system quickly find the data it needs.
Network operations also need optimization. Compression reduces the amount of data sent over the network. Delta compression only transfers changed parts of files.
Caching improves speed for repeated operations. Local caches store frequently accessed data. This cuts down on network requests and disk reads.
Distributed Teams
Version control must support teams spread across different locations. This brings unique scaling challenges. Distributed version control systems shine here. They let each developer have a full copy of the repository. This supports offline work and faster local operations.
But syncing changes can be tricky. Merge conflicts may arise more often with distributed teams. Good communication and clear workflows help avoid issues.
Tools that support code review become more important. They help maintain code quality across distributed teams. Automated tests integrated with version control can catch problems early.
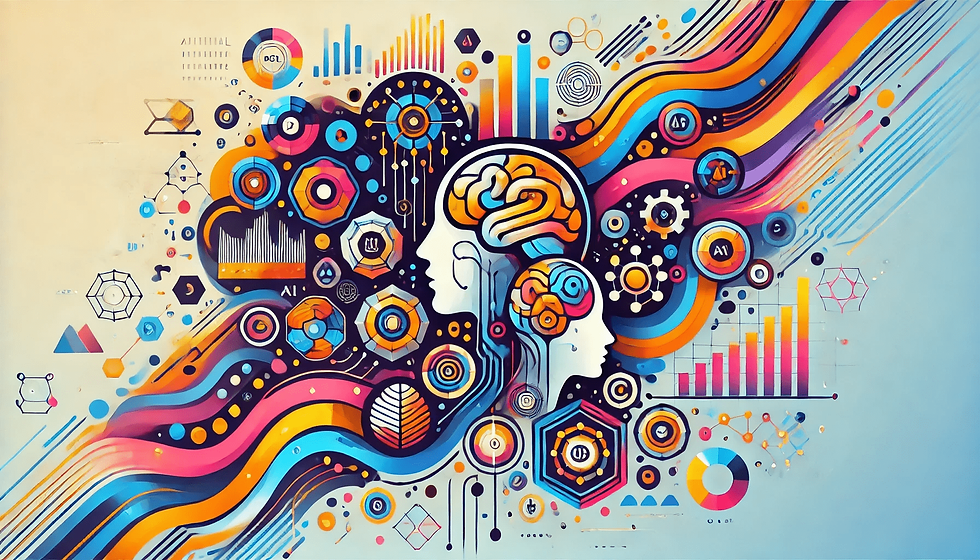
Version Control in Project Management
Version control in project management helps teams track changes, collaborate effectively, and maintain project integrity. It offers tools for managing workflows, monitoring progress, and creating detailed audit trails.
Workflow Management
Version control systems streamline project workflows by enabling teams to work on different parts of a project simultaneously. Team members can create branches to experiment with new ideas without affecting the main project. This agile approach allows for quick iterations and easy integration of successful changes.
Version control tools also help manage code reviews. Developers can submit changes for review before merging them into the main project. This process improves code quality and reduces errors.
Some systems offer built-in task management features. These allow teams to link tasks to specific code changes, making it easier to track work progress.
Tracking Progress
Version control systems provide a clear view of project progress over time. They show who made what changes and when helping managers understand the project's evolution.
Teams can use version control to set milestones and track their completion. This feature is useful for planning releases and managing deadlines.
Version control also helps identify bottlenecks in the development process. By analyzing commit patterns, managers can spot areas that need more resources or attention.
Audit Trails
Version control creates detailed audit trails of all project changes. This record is valuable for compliance, troubleshooting, and understanding project history.
Teams can use audit trails to roll back to previous versions if needed. This ability is helpful when bugs are introduced or when exploring alternative solutions.
Audit trails also support knowledge transfer within teams. New members can review the project's history to understand past decisions and current structure.
Behavior-driven development frameworks often integrate with version control systems. This integration helps teams maintain clear links between project requirements and implemented features.
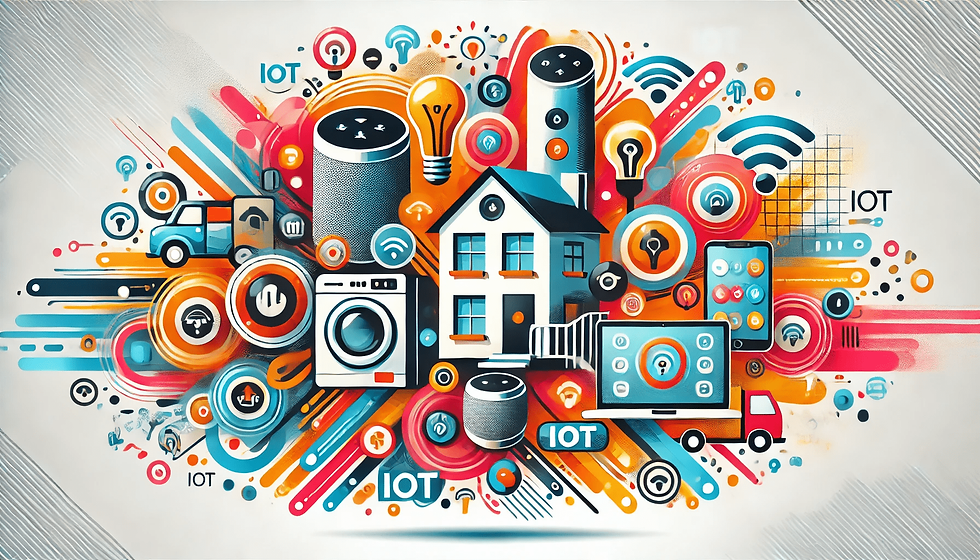
Final Thoughts
Version control is a valuable tool for software developers. It helps teams work together more smoothly and keeps track of changes to code over time. With version control, developers can experiment freely without worrying about breaking things.
Some popular version control systems include Git and Subversion. These tools let multiple people work on the same project at once. They also make it easy to go back to previous versions if needed.
Using version control takes some getting used to, but the benefits are worth it. It can save time, reduce errors, and improve collaboration. Many companies now consider version control a must-have for software projects.
As software keeps evolving, version control will likely become even more important. New features and tools may make it even easier to manage complex codebases. For now, learning the basics of version control is a smart move for any developer.
Frequently Asked Questions
Let’s talk about the most common questions regarding version control.
How does version control enhance team collaboration in software development?
Version control allows multiple developers to work on the same project at once. It lets them share code, merge changes, and avoid conflicts. Team members can see who made what changes and when. This makes it easier to coordinate work and solve problems.
What are the main differences between centralized and distributed version control systems?
Centralized systems have one main repository. All developers connect to it to get and save changes. Distributed systems let each developer have a full copy of the project. This allows for offline work and more flexible workflows.
Can you list common version control systems used in DevOps practices?
Some popular version control systems are:
Git
Subversion (SVN)
Mercurial
Perforce
Git is widely used in DevOps because it's fast and works well with other tools.
What are the advantages of using version control in software project management?
Version control helps manage projects better. It keeps a record of all changes, making it easy to track progress. Managers can see who's working on what and how the project is moving along. It also makes it simpler to plan releases and manage different versions of the software.
How does version control contribute to the history tracking and accountability of changes in software development?
Version control keeps a detailed record of every change made to the code. Each change is linked to the person who made it. This creates a clear history of the project. If something goes wrong, it's easy to find out when and why it happened.
What are the critical features to consider when selecting a version control tool for a software project?
When picking a version control tool, think about:
How easy it is to use
If it works well with your team's size and location
How it handles branching and merging
If it integrates with other tools you use
How well it performs with large projects
The right tool should fit your team's needs and make your work easier.
Disclosure: We may receive affiliate compensation for some of the links on our website if you decide to purchase a paid plan or service. You can read our affiliate disclosure, terms of use, and our privacy policy. This blog shares informational resources and opinions only for entertainment purposes, users are responsible for the actions they take and the decisions they make.
This blog may share reviews and opinions on products, services, and other digital assets. The consumer review section on this website is for consumer reviews only by real users, and information on this blog may conflict with these consumer reviews and opinions.
We may also use information from consumer reviews for articles on this blog. Information seen in this blog may be outdated or inaccurate at times. We use AI tools to help write our content. Please make an informed decision on your own regarding the information and data presented here.
More Articles

Get Brand Exposure
With Smart Advertising
Starter
250$Every monthGreat for startups looking to increase visibility for their brand.Valid for 12 monthsBusiness
1,000$Every monthGreat for mid-size brands looking to increase brand awareness.Valid for 12 monthsEnterprise
6,000$Every monthEstablished businesses looking to collaborate with Digital Products.Valid for 12 months
Stand out and appear in front of people. Advertise to reach a wider audience.
Table of Contents
Disclosure: We may receive affiliate compensation for some of the links on our website if you decide to purchase a paid plan or service. You can read our affiliate disclosure, terms of use, and privacy policy. Information seen in this blog may be outdated or inaccurate at times. We use AI tools to help write our content. This blog shares informational resources and opinions only for entertainment purposes, users are responsible for the actions they take and the decisions they make.